"99 little bugs in the code, 99 little bugs. Take one down, patch it around, 117 little bugs in the code."
In this blog post, we'll explore the significance of Dependency Injection and how it can enhance the quality of your MVC-based C# application.
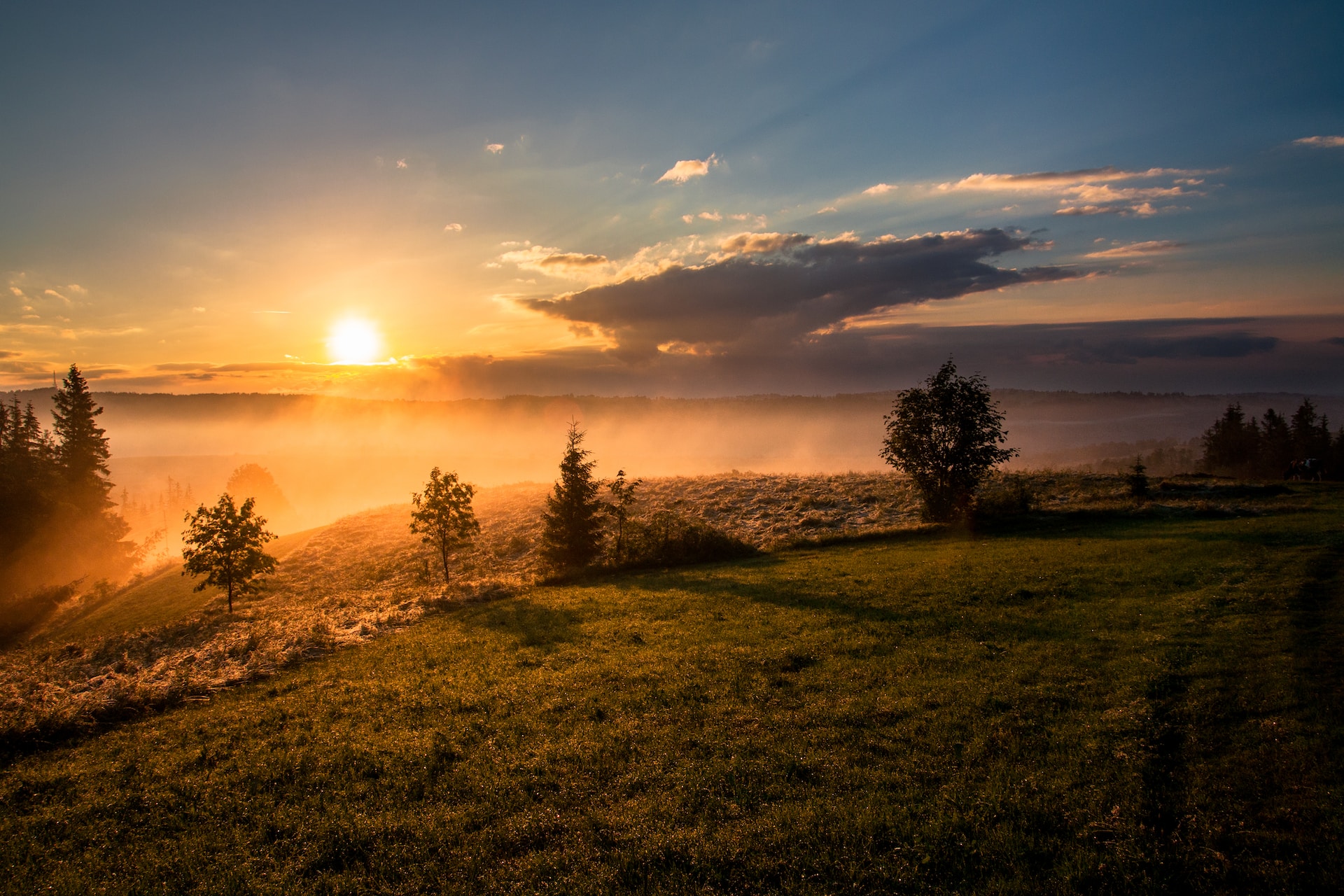
The Issue: Direct Database Access in Controllers
In many MVC applications, you'll find code that directly interacts with the database inside the controllers. While this works for simple apps, it creates problems as your app grows:
Mixed Responsibilities: Controllers become cluttered with both business logic and database queries, making your code hard to understand and maintain.
Testing Challenges: Testing controllers becomes difficult because they are closely tied to the database. It's hard to test them in isolation.
Repeating Code: You might end up duplicating database access code across different controllers, leading to messy and error-prone code.
The Solution: Dependency Injection
Dependency Injection is a way to untangle these issues by:
1. Separating Database Access in a Service Layer
With Dependency Injection, you create a dedicated service layer responsible for all database operations. This separation ensures that controllers only deal with user requests and responses, while the service layer handles data retrieval and manipulation.
For example, instead of having database code in your controller, you'd have something like this:
“ public class ProductService
{ private readonly IDbRepository _repository;
public ProductService(IDbRepository repository)
{ _repository = repository; } ”
2. Making Testing Easier
Dependency Injection allows you to test controllers more effectively. You can replace the real database component with a mock one during testing. This means you can test how your controller behaves without worrying about connecting to an actual database.
For instance:
public class ProductController
{ private readonly IProductService _productService;
public ProductController(IProductService productService)
{ _productService = productService; }
}
3. Reusing Code
By centralizing database access logic in a service layer, you eliminate code duplication across controllers. You can use these service components throughout your application, ensuring a clean and consistent codebase.
Conclusion
Dependency Injection is a valuable technique for improving your MVC C# application's structure and maintainability. By moving database access out of controllers and into a dedicated service layer, you achieve a cleaner separation of responsibilities. This approach leads to cleaner, more adaptable code and makes it easier to create robust and maintainable applications.
0 Comments